Observability 101: Understanding Log Levels: Types, Usage, and Examples
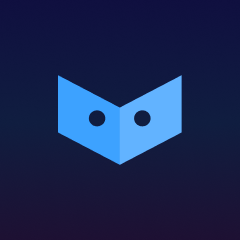
Introduction
Effective logging is crucial for monitoring and maintaining software applications. Logging helps developers and system administrators track the flow of execution, identify issues, and understand application behavior. One of the key aspects of logging is the use of logging levels, which categorize the importance and type of messages being logged. In this blog post, we will explore the different logging levels, their purposes, and best practices for using them, along with examples to illustrate their application.
What are Logging Levels?
Logging levels are predefined categories that indicate the severity or importance of a log message. By assigning a specific level to each log entry, developers can control the granularity of the information captured and filter log messages based on their relevance. This helps in maintaining a balance between the verbosity of logs and their usefulness.
Common Logging Levels
While different logging frameworks might use slightly different names or have additional levels, the following are the most commonly used logging levels:
- DEBUG:some text
- Purpose: Detailed information typically of interest only when diagnosing problems.
- Use Case: Used during development to understand the flow and state of the application.
Example:
python
Copy codelogger.debug("User %s has been authenticated successfully", user_id)
- INFO:some text
- Purpose: Confirm that things are working as expected.
- Use Case: Used to log general information about the application's operation.
Example:
python
Copy codelogger.info("Application started on port %d", port)
- WARNING:some text
- Purpose: Indicate that something unexpected happened, or indicative of some problem in the near future (e.g., ‘disk space low’). The software is still working as expected.
- Use Case: Used to log potentially harmful situations.
Example:
python
Copy codelogger.warning("Disk space is running low: %d%% remaining", disk_space_percentage)
- ERROR:some text
- Purpose: Due to a more serious problem, the software has not been able to perform some function.
- Use Case: Used to log errors that might allow the application to continue running.
Example:
python
Copy code
logger.error("Failed to connect to database: %s", error_message)
- CRITICAL:some text
- Purpose: A serious error, indicating that the program itself may be unable to continue running.
- Use Case: Used to log severe error events that lead to application termination.
Example:
python
Copy code
logger.critical("System is out of memory, shutting down")
How to Use Logging Levels Effectively
- Appropriate Level Selection:some text
- Use DEBUG for granular diagnostic information.
- Use INFO for general operational messages that highlight the progress of the application.
- Use WARNING for messages about potential issues or upcoming problems.
- Use ERROR for logging actual errors that occur during execution but do not halt the application.
- Use CRITICAL for logging serious issues that require immediate attention and might halt the application.
- Consistent Logging:some text
- Ensure consistent use of logging levels across the application to maintain clarity and utility of log messages.
- Contextual Information:some text
- Include relevant context in log messages to make them more informative and useful during debugging and analysis.
- Performance Considerations:some text
- Be mindful of the performance impact of excessive logging, especially at DEBUG level. Disable or reduce logging verbosity in production environments if necessary.
- Log Rotation and Management:some text
- Implement log rotation and management strategies to handle log file sizes and retention policies.
Examples of Logging Levels in Different Scenarios
Web Application Startup:
python
Copy code
import logging
logger = logging.getLogger(__name__)
def start_application(port):
logger.info("Application starting on port %d", port)
try:
# Simulate application start
if port == 0:
raise ValueError("Port number cannot be zero")
except ValueError as e:
logger.error("Error starting application: %s", e)
start_application(8080)
start_application(0)
User Authentication:
python
Copy codedef authenticate_user(username, password):
logger.debug("Authenticating user %s", username)
if username == "admin" and password == "admin":
logger.info("User %s authenticated successfully", username)
else:
logger.warning("Authentication failed for user %s", username)
authenticate_user("admin", "admin")
authenticate_user("user", "wrongpassword")
Database Connection:
python
Copy codedef connect_to_database(connection_string):
logger.info("Connecting to database with connection string: %s", connection_string)
try:
# Simulate database connection
if connection_string == "":
raise ConnectionError("Connection string cannot be empty")
except ConnectionError as e:
logger.critical("Database connection failed: %s", e)
connect_to_database("db_connection_string")
connect_to_database("")
Conclusion
Understanding and effectively using logging levels is essential for maintaining robust and maintainable software applications. By categorizing log messages based on their importance and relevance, developers can ensure that logs provide valuable insights without becoming overwhelming. Using logging levels consistently and appropriately helps in diagnosing issues, monitoring application health, and ensuring smooth operations. Implementing best practices for logging can greatly enhance the ability to troubleshoot and maintain applications, ultimately contributing to more reliable and efficient software systems